[참고포스트]
http://javaking75.blog.me/140187408692
http://javaking75.blog.me/140157710530
http://docs.oracle.com/javase/7/docs/api/java/lang/String.html
[Java] 문자열 비교하기
문자열(String) 객체에 내장된 equals(), equalsIgnoreCase(), compareTo(), compareToIgnoreCase() 메서드로 문자열의 내용을 비교한다.
메서드 | 설명 |
boolean equals(Object obj) | 매개변수로 받은 문자열(obj)과 String인스턴스의 문자열을 비교한다. obj가 String이 아니거나 문자열이 다르면 false를 반환 |
boolean equalsIgnoreCase(String str) | 문자열과 String인스턴스 문자열을 대소문자 구분없이 비교 |
int compareTo(String anotherString) | 문자열을 사전순으로 비교, - 두 문자열이 사전 순으로 같다면 0을 반환 - 대상 문자열 (메서드가 호출된 문자열)이 매개변수로 받은 문자열보다 사전 순으로 앞선 경우 음수를 반환. - 대상 문자열 (메서드가 호출된 문자열)이 매개변수로 받은 문자열보다 사전순으로 뒤질 경우 양수를 반환. |
int compareToIgnoreCase(String str) | 문자열을 사전순으로 비교하되 대소문자 구분없이 비교 |
boolean startWith(String prefix) | 대상 문자열이 매개변수로 받은 접두사(prefix) 문자열로 시작하는지 확인 후 boolean 값을 반환 |
boolean endWith(String suffix) | 대상 문자열이 매개변수로 받은 접미사(suffix) 문자열로 끝나는지 확인한 후 boolean 값을 반환 |
[코드] |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| public class StringCompareExam01 { public static void main(String[] args) { String one = "one"; String two = "two"; String str1 = "one"; String str2 = "Two"; String pieceone = "o"; String piecetwo = "ne"; if ( one.equals(str1) ) { //true System.out.println("String one equals str1 using equals"); } if ( one.equals(two) ) { //false System.out.println("String one equals two using equals"); } if ( two.equals(str2) ) { //false System.out.println("String two equals str2 using equals"); } if ( one == str1 ) { //true, 하지만 연산자 == 는 문자열의 내용을 직접 비교하지 않는다. ( 객체참조비교) System.out.println("String one equals var1 using =="); } if ( two.equalsIgnoreCase(str2) ) { //true System.out.println("String two equals str2 using equalsIgnoreCase"); } System.out.println("Trying to use == on Strings that are pieced together"); //String piecedTogether = pieceone.concat(piecetwo); //문자열 결합 메서드 String piecedTogether = pieceone + piecetwo; if(one.equals(piecedTogether)) { //true System.out.println("The strings contain the same value using equals"); } if(one == piecedTogether) { //false System.out.println("The strings contain the same value using =="); } if (one.compareTo(str1) == 0) { //true System.out.println("One is equal to str1 using compareTo()"); } if ( two.compareToIgnoreCase(str2) == 0) { //true System.out.println("two is equal to str2 using compareToIgnoreCase()"); } } } |
|
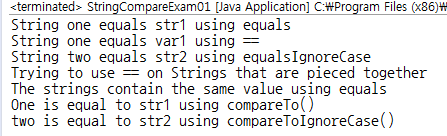 |
http://m.blog.naver.com/javaking75/220487272711
댓글